Table of Contents
Blinking an LED with the CORE MCU Framework PIC16F15313
Let’s be honest, blinking an LED might not be the most exciting project in the world, but there’s something satisfying about seeing that little light turn on and off when you made it happen. It’s like saying, “Hello, world!” to the physical side of programming—proof that the code you wrote is bringing something to life.
In this project, we’re going to blink an LED on a PIC16F15313 using my CORE MCU Framework. It’s a great starting point, and while it might seem simple, mastering the basics is where every great project begins. Ready to get that LED blinking? Let’s dive in!
First Things First: Setting Up the Circuit
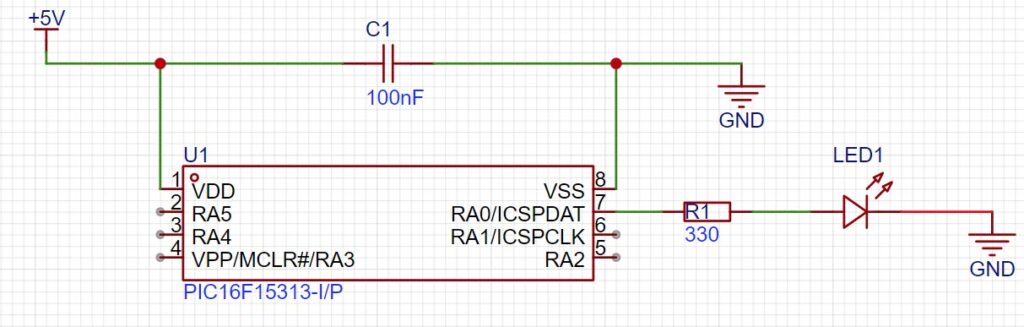
Let’s start by building a straightforward circuit. We’ll be using a PIC16F15313 microcontroller, powered by a 5V DC supply. It’s simple but effective.
The PIC16F15313 is at the heart of our setup, receiving the power it needs to run smoothly.
A 100nF capacitor is connected for decoupling. Think of it as a little “smoothing” tool that keeps the power line steady, preventing unwanted fluctuations.
From there, PortA.0 on the PIC is connected to a 300-ohm resistor, which then drives the LED.
The resistor limits the current flowing through the LED, protecting it from burnout, while ensuring it glows just right. Once connected, it’s all set to blink on command. Simple components working together to create something visible and tangible—that’s the beauty of basic circuits!
Now, Let’s Dive into the Code
Here’s where the magic happens. We’re going to blink an LED using a small piece of code written in C, tailored for the PIC16F15313 microcontroller. This is a simple example, but it’s the foundation for understanding how to control hardware with software—an essential skill when working with microcontrollers.
Below is the code that makes this project come to life:
/****************************************************************************
* Title : Blink LED
* Filename : blink_led.c
* Author : Jamie Starling
* Origin Date : 2024/04/24
* Version : 1.0.0
* Compiler : XC8
* Target :
* Copyright : Jamie Starling
* All Rights Reserved
*
* THIS SOFTWARE IS PROVIDED BY JAMIE STARLING "AS IS" AND ANY EXPRESSED
* OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL JAMIE STARLING OR ITS CONTRIBUTORS BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT,
* STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING
* IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF
* THE POSSIBILITY OF SUCH DAMAGE.
*
*******************************************************************************/
/******************************************************************************
* LICENSED FOR NON-COMMERCIAL USE
* Visit https://jamiestarling.com/corelicense
* for details
*******************************************************************************/
/******************************************************************************
* Includes
*******************************************************************************/
#include "core16F/core16F.h" //Include Core MCU Functions
/******************************************************************************
* Functions
*******************************************************************************/
void main(void)
{
/*Setup*/
/*Initialize for the Core8 System */
CORE.Initialize(); //
/*Set PORTA.0 to Output*/
GPIO.ModeSet(PORTA_0,OUTPUT);
while(1) //Program loop
{
GPIO.PinToggle(PORTA_0); //Toggles LED on PORTA_0
CORE.Delay_MS(1000); //One Second Delay
}
}
/*** End of File **************************************************************/
Breaking Down the Code Step-by-Step
Initialize the Core System: The CORE.Initialize() function sets up the microcontroller’s core settings, getting everything ready for us to control the GPIO pins.
Set Up the Output Pin: GPIO.ModeSet(PORTA_0, OUTPUT); configures the microcontroller’s PORTA.0 pin as an output. This is where we connected the LED in our circuit.
Blinking the LED: The while(1) loop is where the magic happens. Inside this endless loop, we call GPIO.PinToggle(PORTA_0);, which turns the LED on if it’s currently off and off if it’s on.
Adding a Delay: The CORE.Delay_MS(1000); function creates a 1-second delay between each toggle, giving us a steady on-off blinking pattern.
Why This Code Matters
This might seem like a small project, but it’s a powerful demonstration of how code can interact with hardware. By changing a few lines, you can control different pins, adjust the delay, or even create more complex patterns. Every project, no matter how small, is a stepping stone toward mastering microcontroller programming.
Endless Possibilities
With this basic setup, you’re now ready to explore even more possibilities—adding multiple LEDs, creating different patterns, or using inputs to control the blink. Remember, it’s the small steps that build up to big projects! Happy coding!
Core MCU Framework Links
Core MCU Framework Versions : Supported Devices
Have a Project or Idea!?
Seeking Bespoke Technology Solutions?
Contact me today to discover how personalized, high-end tech solutions can meet your unique requirements.