Table of Contents
PIC10F322 Applications: Replacing 555 Timer with PIC10F322 for Basic Astable Circuits
Using the PIC10F322 as a replacement for the 555 timer in creating basic astable circuits provides a flexible and programmable solution. This guide will walk you through setting up an astable circuit with the PIC10F322, including detailed code and explanations.
Overview
In this example, we will generate output pulses on PORTA.0. The microcontroller will run at 16MHz, and the output pulse frequency will be approximately 1.333MHz with a pulse width of roughly 750ns. This setup achieves a 50% duty cycle by incorporating NOP (No Operation) instructions to balance the execution cycles.
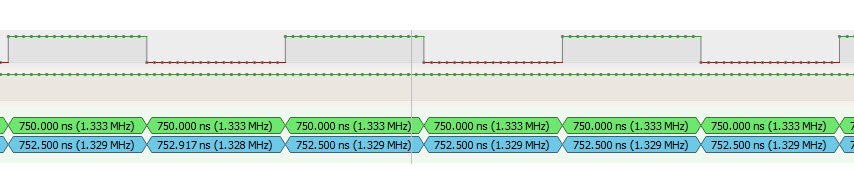
XC8 Code for Basic Astable Circuit
The following XC8 code sets up the PIC10F322 to function as an astable multivibrator, toggling PORTA.0 at a frequency determined by the system clock and the delays implemented.
/*
* File: 555_astable.c
* Author: Jamie Starling - GizoFoundry.com
*
* Created on: September 7, 2021, 7:45 PM
*
* Code/Circuit provided as-is.
*/
#include <xc.h>
#include <stdint.h>
//Device Configuration
#pragma config FOSC = INTOSC // Oscillator Selection
#pragma config BOREN = ON // Brown-out Reset
#pragma config WDTE = OFF // Watchdog Timer
#pragma config PWRTE = ON // Power-up Timer
#pragma config MCLRE = OFF // MCLR Pin Function Select bit->MCLR pin function is digital input, MCLR internally tied to VDD
#pragma config CP = OFF // Code Protection
#pragma config LVP = OFF // Low-Voltage Programming
#pragma config LPBOR = ON // Brown-out Reset Selection bits
#pragma config BORV = LO // Brown-out Reset Voltage Selection
#pragma config WRT = OFF // Flash Memory Self-Write Protection
//Used to calculate the delay time - Change depending on processor Speed
#define _XTAL_FREQ 16000000 //16Mhz
//Prototypes
void setup(void);
void main(void)
{
setup();
while(1)
{
LATAbits.LATA0 = 1; //Put A.0 High
/*We call an assembly insuctgion here NOP - no operation it burns 1 CPU cycle
*We add two - because once the code hits the end, it will execute an ASM JUMP which takes two cpu cycles to complete
*this gives us a nice square 50% dutycycle wave at 750ns high and low */
NOP();
NOP();
//Add Delay here if you need something different
LATAbits.LATA0 = 0; //Put A.0 low
//AND Add the same Delay here if you need something different for 50%
}
}
void setup(void)
{
//Disable analog for A0, set as Output, Set to Low
ANSELAbits.ANSA0 = 0;
TRISAbits.TRISA0 = 0; //A0 Output
LATAbits.LATA0 = 0; //Put A0 low
//Set the System Clock - You can change this to match the setting you are looking for
OSCCONbits.IRCF = 0b111; //Set System Clock to 16Mhz FOSC/4 = 4Mhz
}
Explanation of the Code
- Device Configuration:
- The configuration bits set the various hardware options such as oscillator selection, brown-out reset, and power-up timer settings.
- Setup Function:
- Disables the analog function on PORTA.0 and sets it as an output.
- Configures the system clock to 16MHz.
- Main Loop:
- The main loop toggles PORTA.0 high and low, with two NOP instructions between each toggle to balance the instruction cycles and achieve a 50% duty cycle.
Adjusting the Pulse Width and Frequency
To achieve different pulse widths or frequencies, you can adjust the number of NOP instructions or add delays. Here’s how to calculate the required number of instructions for a desired pulse width:
- Instruction Cycle Time: At 16MHz, the instruction cycle is 4MHz (250ns per instruction).
- Desired Pulse Width: For a 1μs pulse width, you need 1μs / 250ns = 4 instruction cycles.
Summary
Replacing the 555 timer with a PIC10F322 for basic astable circuits provides greater flexibility and precision. This guide covers the necessary circuit setup and provides a detailed explanation of the XC8 code to create an astable multivibrator. By leveraging the capabilities of the PIC10F322, you can easily adjust the frequency and duty cycle to meet your specific needs.
Have a Creative or Technical Project in Mind?
Looking for guidance, insights, or a fresh perspective on your technical or creative journey? Or just somebody to chat with?
Reach Out
jamie@jamiestarling.com