Color Image Arrays for image processing.
Up to this point we have been dealing with Black and White images or Gray Scale images – that can be represented by a 2d array. Array(height, width)
In which each element in the array represents one pixel and the level of gray, with 0 being black and 255 being white.
A color image is made up of a combination of three color channels, RGB – Red, Green, Blue.
In order to represent the image in an array, requires a 3d array. Array(height, width, channel)
Channel also can be refered to as the block size when talking about arrays.
Each elements represents the brightness of the color channel, and each pixel is then made up of the three channels.
For a given pixel you end up with 256x256x256 (the possible color levels) – which yields 16,777,216 possible color combinations. I am not sure, but I think that is more than Crayola Crayons creates.
Something to consider is the memory requirements for a 3d array. Given our previous example, a black and white – gray scale image, (200×100) takes up 20k (roughly) of RAM. For a color one, (200x100x3) will consume about 60K of RAM.
Working with color images takes more RAM, but you will also need to process the additional blocks of color information compared to a gray scaled image.
Lets make a quick color image – it is better to see it in action then me try to explain it.
The following Python code will create a 5x4x3 array of a image with a Red Line, Green, Line, Blue Line, White Line, Black Line.
Each pixel is grouped into elements of three [R,G,B] – the first number is the Red Value, the Second Green, and the Last is Blue.
It is very similar to our black and white code, except it is a 3d array.
#%%
import matplotlib.pyplot as plt
import numpy as np
# 5x4x3 Color - Line 1 Red, Line 2, Green, Line 3 Blue, Line 4 White, Line 5 Black
imgArray = np.array([[[255, 0, 0],[255, 0, 0],[255, 0, 0],[255, 0, 0]],
[[0, 255, 0],[0, 255, 0],[0, 255, 0],[0, 255, 0]],
[[0, 0, 255],[0, 0, 255],[0, 0, 255],[0, 0, 255]],
[[255, 255, 255],[255, 255, 255],[255, 255, 255],[255, 255, 255]],
[[0, 0, 0],[0, 0, 0],[0, 0, 0],[0, 0, 0]]])
# Using matplot to load the array into their image display function
plt.imshow(imgArray)
# Shows the Image
plt.show()
You will get this as the output.
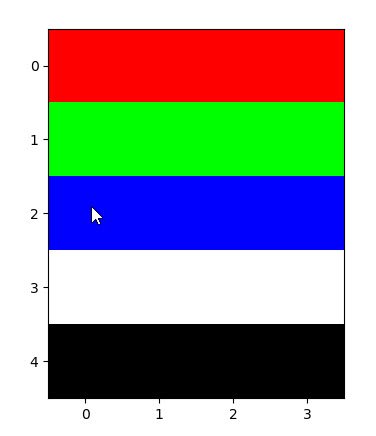
Play around with changing the colors up.
See the Image Processing Table of Contents
Have a Project or Idea!?
Seeking Bespoke Technology Solutions?
Contact me today to discover how personalized, high-end tech solutions can meet your unique requirements.