In the last post we took a look at averaging the RGB channels to produce a gray scale image. It does require some math division to get the result.
If you need a way that is even simpler and faster than averaging – we can extract the values from one of the color channels only. This method requires no calculations. All it does is pick a single channel and makes that the grayscale value
Some low-end cameras uses this as the process of producing black and white images.
The green channel is usually the channel of choice when using this option. However, some intresting results can be gained from choosing the other channels as well.
For example, if you where looking for red colored items, perhaps the red channel would be the one to work with, as it would bring objects that contain high levels of red toward white in the gray scale image.
Any way – lets see it in action..
Lets take the image below of 4 balls, Red, Green, Blue, Yellow and see what happens when we process a single channel only.
For the code, uncomment which channel you want to extract.
The Input Image
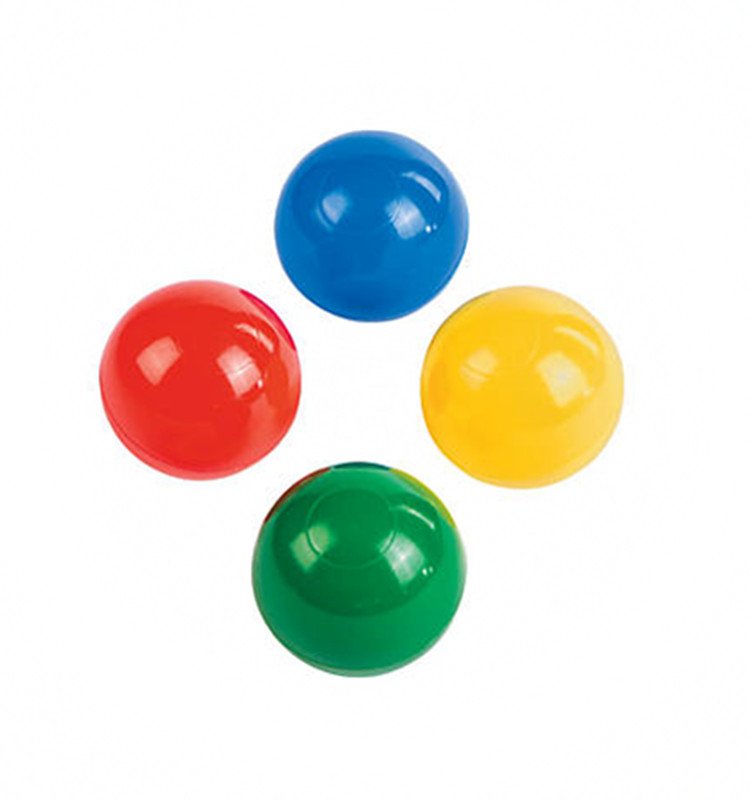
The Code
#%%
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
# Image File we are loading
imageFile = r"D:\temp\rgbballs.jpg"
# Pillow Image Object
inputImage = Image.open(imageFile)
# Convert To Numpy Array
imageArray = np.array(inputImage)
#%%
# From the Array get the Image(array) size
imageHeight = imageArray.shape[0]
imageWidth = imageArray.shape[1]
# Create Empty Array to store the gray scale image
imageArrayGrayScale = np.empty([0,imageWidth], dtype=np.uint8)
# Set the counters so we can move through the array
imageHeightCurrentPosition = 0
imageWidthCurrentPosition = 0
# Go into the Array, and for each row, extract the data out
while imageHeightCurrentPosition < imageHeight:
# Create Empty list to hold the new gray scale row data
imageRowList = []
# For each column, extract the RGB values for each dimension.
while imageWidthCurrentPosition < imageWidth:
redValue = int(imageArray[imageHeightCurrentPosition,imageWidthCurrentPosition,0])
greenValue = int(imageArray[imageHeightCurrentPosition,imageWidthCurrentPosition,1])
blueValue = int(imageArray[imageHeightCurrentPosition,imageWidthCurrentPosition,2])
# Gray scale channel extraction
# Uncomment for the Red Channel
grayValue = np.uint8(redValue)
# Uncomment for the Green Channel
#grayValue = np.uint8(greenValue)
# Uncomment for the Blue Channel
#grayValue = np.uint8(blueValue)
# Add the gray scale value to our list
imageRowList.append(grayValue)
# Move to the next column
imageWidthCurrentPosition += 1
# finally we store the generated list into the imageArray
imageArrayGrayScale = np.append(imageArrayGrayScale, np.array([imageRowList]), axis=0)
# Increment the row we are working on to move to the next
imageHeightCurrentPosition += 1
# Set our column position back to 0
imageWidthCurrentPosition = 0
# Set the display size to display full image
# Using matplot to load the array into their image display function
plt.figure(figsize = (imageHeight/100,imageWidth/100))
plt.imshow(imageArrayGrayScale)
# We need to make sure matplot knows it is a black and white image
plt.gray()
# Shows the Image
plt.show()
The Results
Red Channel Only
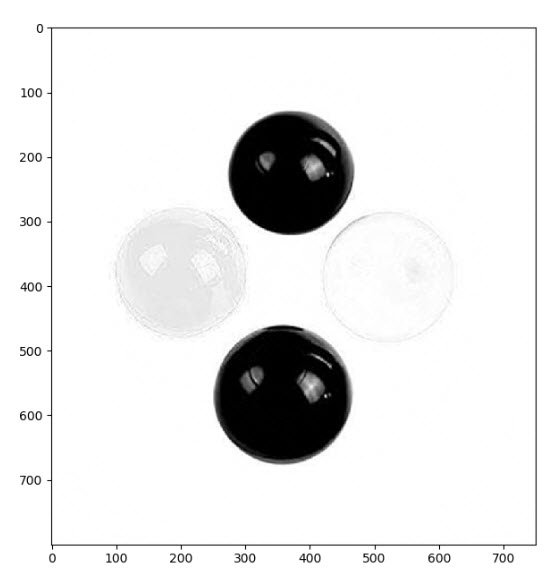
Green Channel Only
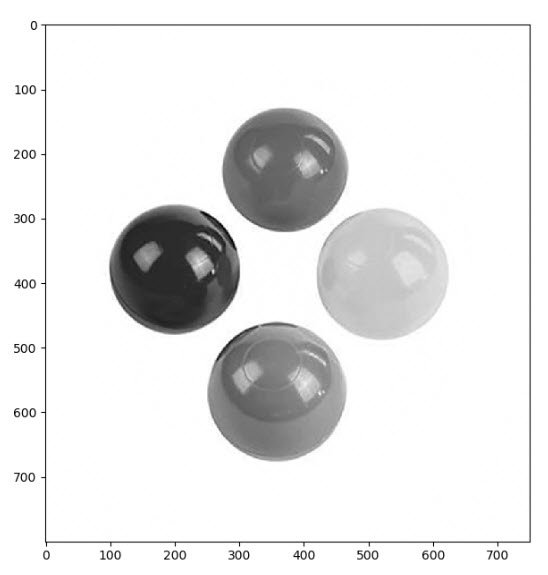
Blue Channel Only
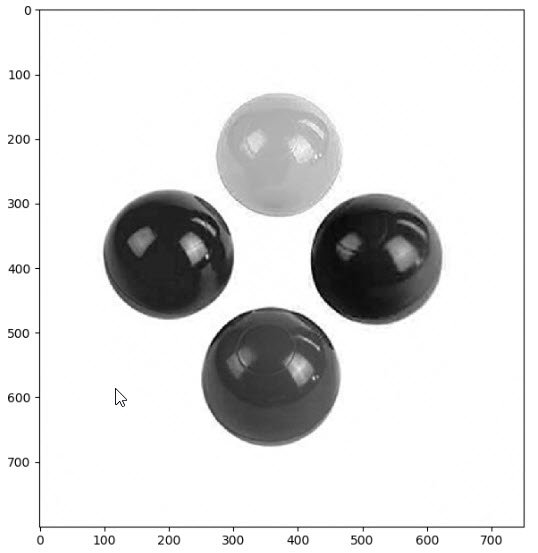
See the Image Processing Table of Contents
Have a Project or Idea!?
Seeking Bespoke Technology Solutions?
Contact me today to discover how personalized, high-end tech solutions can meet your unique requirements.