Histogram for a Gray Scale Image – Using Python.
What is an image histogram – it is a profile of the occurrences of each gray level present in an image.
In the visual example below – we are using a bar graph to visualize it. It starts at 0 and goes to 255, each vertical bar represents the number of times the corresponding gray level occurred in the image.
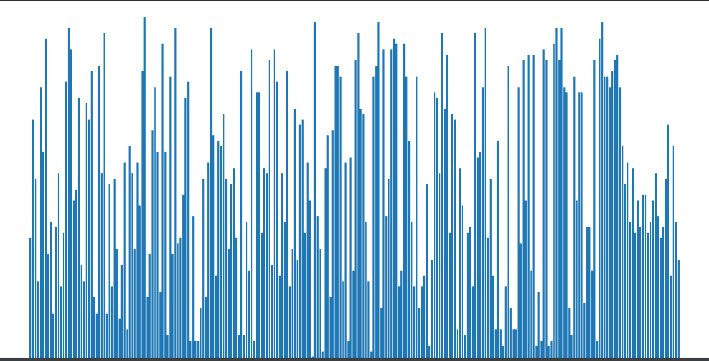
Sp, what are histograms used for? They can help determine if an image is being captured correctly, they can also be used for help select thresholds for object detection. Objects will tend to have similar gray levels.
They are also used to equalize an image. We will get into that next post.
For now here it the Python code for generate a histogram. Much of it is the same code we built upon in previous posts.
The histogram section, moves though the gray scale array, and generates a count for each gray value.
#%%
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
# Image File we are loading
imageFile = r"D:\temp\thecats.jpg"
# Pillow Image Object
inputImage = Image.open(imageFile)
# Convert To Numpy Array
imageArray = np.array(inputImage)
#%%
# From the Array get the Image(array) size
imageHeight = imageArray.shape[0]
imageWidth = imageArray.shape[1]
# Create Empty Array to store the gray scale image
imageArrayGrayScale = np.empty([0,imageWidth], dtype=np.uint8)
# Set the counters so we can move through the array
imageHeightCurrentPosition = 0
imageWidthCurrentPosition = 0
# Go into the Array, and for each row, extract the data out
while imageHeightCurrentPosition < imageHeight:
# Create Empty list to hold the new gray scale row data
imageRowList = []
# For each column, extract the RGB values for each dimension.
while imageWidthCurrentPosition < imageWidth:
redValue = int(imageArray[imageHeightCurrentPosition,imageWidthCurrentPosition,0])
greenValue = int(imageArray[imageHeightCurrentPosition,imageWidthCurrentPosition,1])
blueValue = int(imageArray[imageHeightCurrentPosition,imageWidthCurrentPosition,2])
# Gray scale average is the average of the three colors RGB
grayValue = np.uint8((redValue + greenValue + blueValue)/3)
# Add the gray scale value to our list
imageRowList.append(grayValue)
# Move to the next column
imageWidthCurrentPosition += 1
# finally we store the generated list into the imageArray
imageArrayGrayScale = np.append(imageArrayGrayScale, np.array([imageRowList]), axis=0)
# Increment the row we are working on to move to the next
imageHeightCurrentPosition += 1
# Set our column position back to 0
imageWidthCurrentPosition = 0
# Set the display size to display full image
# Using matplot to load the array into their image display function
plt.figure(figsize = (imageHeight/100,imageWidth/100))
plt.imshow(imageArrayGrayScale)
# We need to make sure matplot knows it is a black and white image
plt.gray()
# Shows the Image
plt.show()
#%% - Histogram
# Set the counters so we can move through the array
imageHeightCurrentPosition = 0
imageWidthCurrentPosition = 0
# Go into the Array, and for each row, extract the data out
while imageHeightCurrentPosition < imageHeight:
# Create Array to hold the histogram data
imageHistogramData = np.empty([256], dtype=np.uint8)
# For each column, extract the Gray values
while imageWidthCurrentPosition < imageWidth:
grayValue = int(imageArrayGrayScale[imageHeightCurrentPosition,imageWidthCurrentPosition])
# Create a histogram where each element of the array contains the count of the gray value.
imageHistogramData[grayValue] += 1
# Move to the next column
imageWidthCurrentPosition += 1
# Increment the row we are working on to move to the next
imageHeightCurrentPosition += 1
# Set our column position back to 0
imageWidthCurrentPosition = 0
# Take a look at what we have.
fig = plt.figure(figsize = (10, 5))
ax = fig.add_axes([0,0,1,1])
ax.bar(range(256),imageHistogramData)
plt.show()
See the Image Processing Table of Contents
Need a Creator Alchemist on Your Project?
I’m available for hire—consulting, systems design, embedded tech, creative problem-solving, or anything in between.
If you’ve got a project that needs someone who can think it through and build it out, I’m interested.
👉 Let’s Talk
jamie@jamiestarling.com