Blinking a LED on a MCU is much like a “Hello World” program.
Here is the XC8 Code and circuit to Blink an LED with a PIC10F322 Micro-controller. The LED is hooked up to PortA0.
The code will blink an LED on PortA.0 ever second.
The Circuit
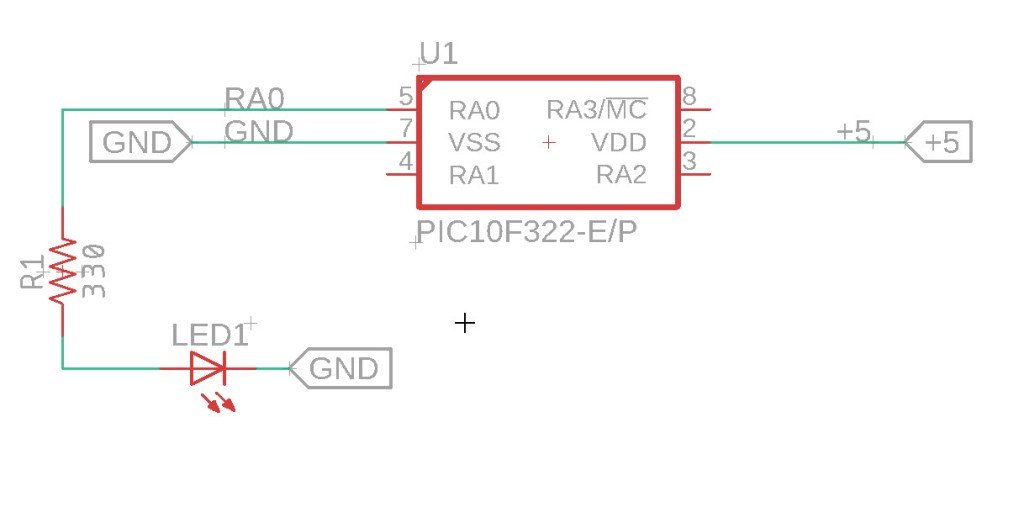
The Code
/*
* File: blink_led.c
* Author: Jamie Starling - GizoFoundry.com
*
* Created on: September 1, 2021, 9:45 AM
*
* Code provided as-is.
*/
#include <xc.h>
#include <stdint.h>
//Device Configuration
#pragma config FOSC = INTOSC // Oscillator Selection
#pragma config BOREN = ON // Brown-out Reset
#pragma config WDTE = OFF // Watchdog Timer
#pragma config PWRTE = ON // Power-up Timer
#pragma config MCLRE = OFF // MCLR Pin Function Select bit->MCLR pin function is digital input, MCLR internally tied to VDD
#pragma config CP = OFF // Code Protection
#pragma config LVP = OFF // Low-Voltage Programming
#pragma config LPBOR = ON // Brown-out Reset Selection bits
#pragma config BORV = LO // Brown-out Reset Voltage Selection
#pragma config WRT = OFF // Flash Memory Self-Write Protection
//Used to calculate the delay time - Change depending on processor Speed
#define _XTAL_FREQ 8000000 //8 MHz (default after Reset)
//Prototypes
void setup (void);
void blink_led(void);
//Main Code Entry
void main(void)
{
setup(); //Device Setup
while(1) //Loop Here forever and do blink_led
{
blink_led();
} //End of while
} //End of Main
void setup (void)
{
ANSELAbits.ANSA0 = 0; //Disable Analog
//Set Pin 0 PortA.0 as output
TRISAbits.TRISA0 = 0;
}//End of setup
void blink_led(void)
{
//Set PortA.0 High (turn on LED)
LATAbits.LATA0 = 1;
//Wait 1 second - delay macro is in ms so 1000ms = 1 second
__delay_ms(1000);
//Set PortA.0 Low (turn off LED)
LATAbits.LATA0 = 0;
//Wait 1 second
__delay_ms(1000);
}//End of blink_led
Have a Project or Idea!?
I am Available for Freelance Projects
My skills are always primed and ready for new opportunities to be put to work, and I am ever on the lookout to connect with individuals who share a similar mindset.
If you’re intrigued and wish to collaborate, connect, or simply indulge in a stimulating conversation, don’t hesitate! Drop me an email and let’s begin our journey. I eagerly anticipate our interaction!