Here is a little fun. Some Python code that will take a text file and turn it into an image.
When I say, turn it into an image – I don't mean it will read the text file and make some image from it that we could look at and say… oh the ocean.
What it does it convert the characters into their ASCII number, makes an array, and displays that array.
With that in mind – you could take an image – convert that into a series of ASCII characters, write it out to a text file – then read it back in and BOOM you would have that image.
However depending on what you are doing a MIME would be a better choice.
But in any event, the code does a good job making noise image.
#Reads in a text file and then displays it has an image.
#%%
import matplotlib.pyplot as plt
import numpy as np
# Input Text File
inputTextFile = r"D:\temp\text.txt"
# Define the size of the numpy array
sizeHeight = 600
sizeWidth = 800
# Create an empty numpy array, with 0 rows, and the width we need.
imageArray = np.empty([0,sizeWidth])
# Function to read a text file and return the contents
def returnTextFileContent(file):
encoding_format = "utf-8"
textDataFile = open(file, mode="r", encoding=encoding_format)
textContent = textDataFile.read()
textDataFile.close()
return textContent
# Read Text File - data in textData var
textData = returnTextFileContent(inputTextFile)
# Check the length of the text file - it needs to be larger than the array or image size
if len(textData) < (sizeHeight * sizeWidth):
print("Text File to short")
exit()
# starting the process of converting the text into ascii numbers and appending them to the array
# var we store the current line we are processing
lineCounter = 0
# we process until we complete the number of vertical lines required
while lineCounter < sizeHeight:
# we grab the sizeWidth from the larger text file
textLine = textData[(lineCounter * sizeWidth):(lineCounter * sizeWidth + sizeWidth)]
# create empty list to split the chars
textList = []
textList[:0] = textLine # do the split
# create another list to store the ascii values of the chars
asciiList1 = []
# alternate over each char in the text list, convert to ascii and store in asciList
for c in textList:
asciiList1.append(ord(c))
# finally we store the generated list into the imageArray
imageArray = np.append(imageArray,np.array([asciiList1]),axis=0)
# update the line counter
lineCounter += 1
# Set the display size to display full image
# Using matplot to load the array into their image display function
plt.figure(figsize = (sizeHeight/100,sizeWidth/100))
plt.imshow(imageArray, aspect='auto')
# We need to make sure matplot knows it is a black and white image
plt.gray()
# Shows the Image
plt.show()
Example output of text to image
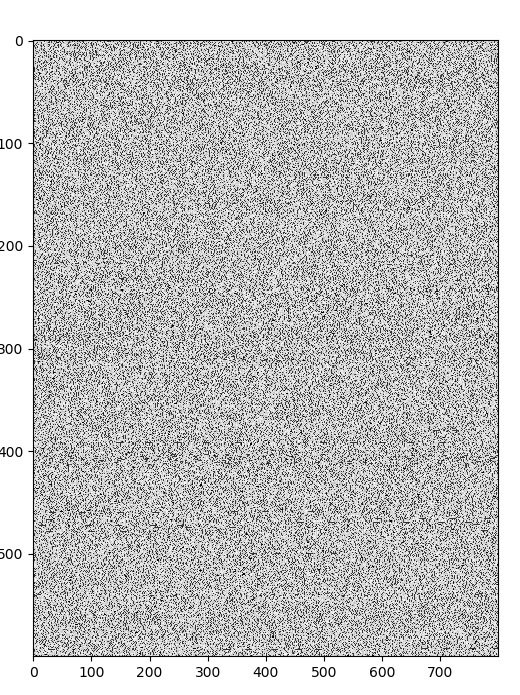
See the Image Processing Table of Contents
Have a Project or Idea!?
Seeking Bespoke Technology Solutions?
Contact me today to discover how personalized, high-end tech solutions can meet your unique requirements.