As you recall – a black and white image can be represented by a 2d array.
The below Python code takes a 2 x 5 array (checker board pattern) and using matplot converts it into an image we can see.
#%%
import matplotlib.pyplot as plt
import numpy as np
# 2d Image Array with data 2 x 5 - Checker Board
imgArray = np.array([[255, 0, 255, 0, 255],
[0, 255, 0, 255, 0]])
# Using matplot to load the array into their image display function
plt.imshow(imgArray)
# We need to make sure matplot knows it is a black and white image
plt.gray()
# Shows the Image
plt.show()
What you get is an output that looks like this.
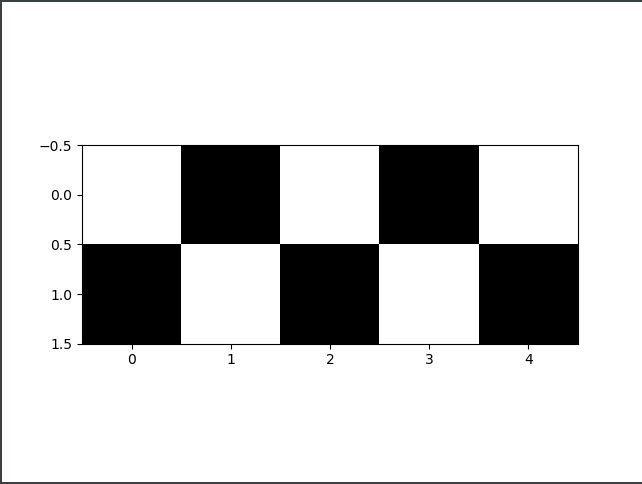
Each element in the array represents a single pixel. Where the element has a value of 0, the pixel will be black. Where the value is 255, the pixel will be white.
Any value in between 0 and 255 will generate a level of gray.
Making something – The Smiley Face
Using the same idea – lets create a 7×7 array that will output a smile face.
#%%
import matplotlib.pyplot as plt
import numpy as np
# 2d Image Array with data 7 x 7 - Smile Face
imgArray = np.array([[255, 255, 255, 255, 255, 255, 255],
[255, 0, 255, 255, 255, 0, 255],
[255, 255, 255, 255, 255, 255, 255],
[255, 255, 255, 255, 255, 255, 255],
[255, 0, 255, 255, 255, 0, 255],
[255, 255, 0, 0, 0, 255, 255],
[255, 255, 255, 255, 255, 255, 255]])
# Using matplot to load the array into their image display function
plt.imshow(imgArray)
# We need to make sure matplot knows it is a black and white image
plt.gray()
# Shows the Image
plt.show()
Run the code and you will get
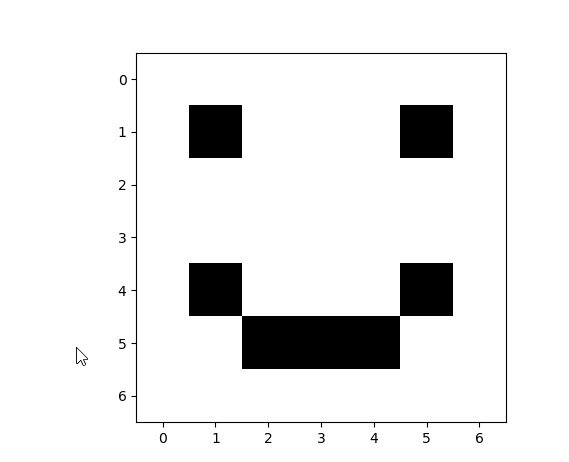
As we can see. images are nothing more then 2d arrays – there is nothing magical about them. This is an important fact to remember – as anything we want to do with them just requires some math.
One more fun Python project after this one – convert a text document into an image.